Busting the Myth: Writing JavaScript Takes Forever!
Coding in JavaScript (or any coding language, for that matter) can be tedious. If you’ve ever had to spend all day scrolling through a thousand lines of code looking for occurrences of a variable or function reference, you know what I mean. However, it’s not terribly difficult to streamline your coding habits to make both the writing AND maintenance of JavaScript much easier and faster!
Below, I’ll go over four easy-to-adopt habits that will cut your coding time down significantly. These small tricks are very helpful to immediately improve your productivity and are great habits to pick up.
1. Use the document.getElementById Shortcut
Photo by Unhindered by Talent
If you write JavaScript, you’re probably pretty familiar with document.getElementById. It’s one of the most useful methods in JavaScript, but its name is awfully long, easy to typo, and painfully tedious to type and retype over and over again.
However, taking a page from the Prototype library, we can write a very quick function to shortcut document.getElementById:
[sourcecode language='javascript']$(id) {
return document.getElementById(id);
}[/sourcecode]
Now a call to document.getElementById(“myElement”); becomes $(“myElement”);
Nice and easy, and it’ll save a ton of time in longer scripts.
NOTE: Using this function will incur a slight performance hit. However, unless you’re splitting milliseconds on your site, it won’t be noticeable.
2. Keep It Variable
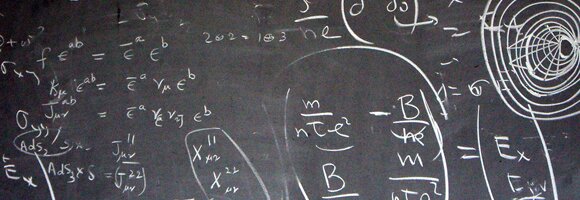
Using functions is a really basic tip, and I hope that everyone reading this already understands the value of encapsulating chunks of relevant code into functions for easy reuse. If you haven’t started using functions yet, then, dude, you’re missing out! You can read a great tutorial on getting started with functions over at w3schools.com.
I’d like to take those functions one step further and stress the importance of keeping your functions completely variable. That means writing a function that accomplishes the task it’s designed to perform with as few hard-coded elements as possible, and storing all site-specific information in variables that will be passed to the function.
Take this example for instance: let’s say you’re writing a script that will validate the input of a comment form to make sure the user entered their name. It might look something like this (if you’re using that fancy-schmancy document.getElementById shortcut):
function validateForm() {
// Check that "name" isn't blank
if ( $("myForm").elements["name"].value == '' ) {
alert('Please fill out all required fields!');
return false;
}
// Submit the form if the field has a value
else
return true;
}
That works just fine, but it’ll have to be edited for another site that needs the exact same feature. And what if you need to validate multiple elements? You’ll end up with a redundant call to validate the next field. That’s a lot of code that’ll have to be edited every time you make a change to the HTML or implement your script on a new site.
To make your code portable, and therefore much faster and easier to reuse, you should make sure your functions rely completely on variables. Let’s see what we can do with the function we just wrote:
function validateForm(formId, fieldId) {
if ( $(formId).elements[fieldId].value == '' ) {
alert('Please fill out all required fields!');
return false;
}
else
return true;
}
At first glance, it might not seem like that makes a huge difference, but when you go to reuse your code, now all you have to add is this:
var formId = "myForm";
var fieldId = "name";
Having all your variables grouped like that, instead of tucked inside a function that might be hidden away in an include file somewhere, will save a lot of headaches, and will ultimately save you a ton of wasted time.
3. Get Comfortable with Arrays and Loops
Photo by Unhindered by .bullish
Continuing with our above example, validating multiple fields would require multiple function calls, which could be a cumbersome task, and, again, could become a huge maintenance nightmare down the road.
To solve this issue, we can employ one of JavaScript’s handy loop constructs. A for loop is a really easy way to execute a command or a set of commands on multiple elements (stored in an array). One particular way of using the for loop is to declare a variable, “x”, without a value, then run the loop as follows:
var x;
for (x in myArray) {
// Do stuff...
}
What this accomplishes, in plain English, is essentially, “For however many elements are in this array, execute the following commands on each one.”
Once you get the hang of how this construct works, it becomes an incredibly useful (and time-saving!) tool.
Let’s look at how we could apply a for loop to make our validateForm() function handle multiple fields:
function validateForm(formId, fieldArray) {
var x;
for ( x in fieldArray ) {
if ( $(formId).elements[fieldArray[x]].value == '' ) {
alert('Please fill out all required fields!');
return false;
}
}
return true;
}
With barely any extra JavaScript, we’ve now created a very portable function that will check multiple fields inside a form to make sure they’re not empty. And all we need to do to customize the script for a new site is this:
var formId = "myForm";
var fieldArray = new Array("name","email","comment");
How easy is that?! You’ll never have to write a validation script again! And if you update it in the future? As long as you don’t change the information needed for the script to run, you can just copy and paste the new function (or drag the updated include file onto the site directory).
Portable scripts to handle common site needs can save hours, and they don’t take too much longer to code than their messier, non-portable counterparts!
4. Abstraction!
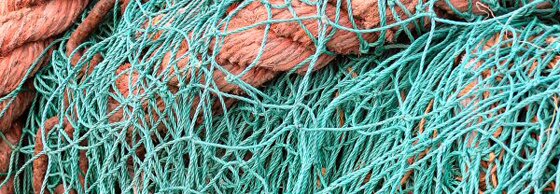
Another great way to cut down on tedious coding is the concept of separating your JavaScript from your HTML markup. The reason this saves time should be obvious, but just in case you’re not seeing my point: let’s say we add our new validation function to a newsletter signup form. That newsletter signup form is on all 28 pages of our client’s site, and you have to make a change. Every form submit button is coded like this:
Finding that snippet of code to edit on 28 pages could prove to be a huge time-suck. Avoiding that, however, is pretty simple. We can simply add the following script in a file that can be included on all pages:
if ( $(formId) )
$(formId).onsubmit = function() { return validateForm(formId, fieldId); }
This allows us to keep our button nice and clean as well:
By lifting the JavaScript out of our markup, we’ve eliminated some of the tediousness out of code maintenance, and with clever script placement, you can consolidate all of your maintenance into one central location.
Conclusion
Even if you only write JavaScript as a hobby, it’s worth it to spend an extra few seconds to optimize your code for easy maintenance and reuse. Many of the scripts I use most often were originally written with no intention of needing them again, and it’s been very convenient to just have them on hand, rather than having to rewrite the script and/or spend an hour or two editing it to suit my purposes.
How do you streamline your coding practice? Share your favorite time-saving tips in the comments!
{ 10 comments… read them below or add one }
A lot of people go through a phase where they use Prototype mostly for the bling function.
Here’s what you want:
$ = document.getElementById;
@Paul
Much simpler! I like it!
Ваш поÑÑ‚ мне очень интереÑен и Ñ Ð±Ñ‹ хотел получить больше информации по Ñтой теме в полÑедующих ваших поÑтах. СпаÑибо за то что вы еÑть. Жду продолжениÑ.
Да Ñто важно, но не тк что бы об Ñтом пиÑать в блоге. Ðто моем мнение, вы не ÑоглаÑны?
This is hardly about efficiency… This is rather just beginner’s guide to programming basics.
raf sistemleri
It’s useful. Thanks so much.
Some of this was useful, however, why not write up an actual efficiency guide with comparisons of before and after, thanks
Very useful and well written tips. Specially the use of array in validation.
I’ve been exploring for slightly to get a good
quality articles or blog posts on this sort of house .
Exploring in Yahoo I at last located this website.
Reading this info So i’m satisfied to exhibit that I’ve an incredibly good uncanny feeling I discovered exactly the things i needed. I lots indubitably is likely to make sure to usually do not disregard this website and present it a peek regularly.
Woh I your posts, saved to favorites! .
{ 1 trackback }